Multi-session applications
Learn how to configure and work with multi-session applications
Overview
One of the most powerful features that Clerk provides out of the box is multi-session applications.
A multi-session application is an application that allows multiple accounts to be signed-in from the same browser at the same time. The user can switch from one account to another seamlessly. Each account is independent from the rest and has access to different resources. It's most clearly explained by the default <UserButton /> below.
Note that you can switch which account is active, add additional accounts, and manage each account independently. To enable a multi-session instance, you need to handle the following scenarios:
- Switching between different accounts
- Adding new accounts
- Signing out from one account, while remaining signed in to the rest
There are two main ways to handle all the above: using Clerk Components or using a custom flow.
Looking for more information on session management? Check out our detailed guide.
Before you start
- You need to create a Clerk Application in your Clerk Dashboard. For more information, check out our Set up your application guide.
- You need to install Clerk React or ClerkJS to your application.
Configuration
The first thing you need to do is to enable the multi-session feature in your Clerk instance.
From the Clerk Dashboard, select your application and instance, navigate to Sessions and choose Multi-session handling.
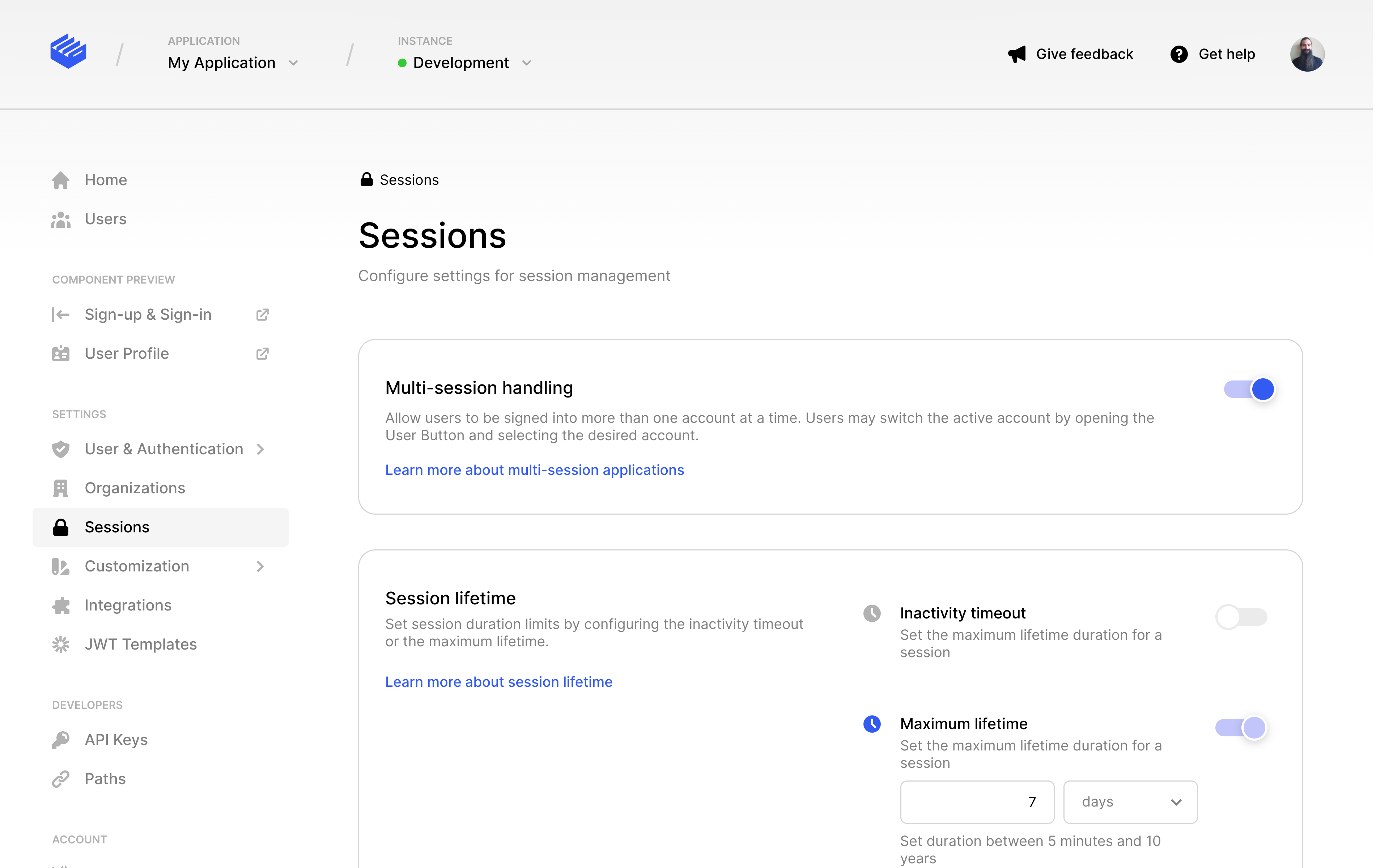
That's all you have to do! Now you have a multi-session application!
Using Clerk Components
The easiest way to add multi-session features to your application is by using the <UserButton /> component.
This component has buttons add a new account, switch between accounts and sign out from one or all accounts.
1import { UserButton } from "@clerk/clerk-react";23const Header = () => {4return (5<header>6<h1>My application</h1>7<UserButton />8</header>9);10};
1<html>2<body>3<header>4<h1>My application</h1>5<div id="user-button"></div>6</header>78<script>9const el = document.getElementById("user-button");10// Mount the pre-built Clerk UserButton component11// in an HTMLElement on your page.12window.Clerk.mountUserButton(el);13</script>14</body>15</html>
Note that you don't need to pass any special options to the UserButton component. For more details on all available options, including customization options, please visit the <UserButton /> guide.
Custom flow
In case you want more customization, and you prefer full control over your UI, you can use Clerk's SDKs.
Active session/user
1import { useClerk } from "@clerk/clerk-react";23// Getting the active session and user4const { session: activeSession, user: activeUser } = useClerk();
1// Getting the active session2const activeSession = window.Clerk.session;34// Getting the active user5const activeUser = window.Clerk.user;
Switching sessions
1import { useClerk } from "@clerk/clerk-react";23const { client, setSession } = useClerk();45// You can retrieve all the available sessions through the client6const availableSessions = client.sessions;78// Use setSession to set the active session.9setSession(availableSessions[0].id);
1// You can retrieve all the available sessions through the client2const availableSessions = window.Clerk.client.sessions;34// Use setSession to set the active session.5window.Clerk.setSession(availableSessions[0].id);
Adding sessions
To add a new session, simply link to your existing sign in flow. New sign ins will automatically add to the list of available sessions on the client.To create a sign in flow, please check one of the following popular guides:
For more information on how Clerk's sign in flow works, checkout our detailed sign in guide.
Sign out active session
This version of sign out will deactivate the active session. The rest of the available sessions will remain intact.
1import { useClerk } from "@clerk/clerk-react";23const { signOutOne } = useClerk();45// Use signOutOne to sign-out only from the active session.6await signOutOne();
1// Use signOutOne to sign-out only from the active session.2await window.Clerk.signOutOne();
Sign out of all sessions
This request will deactivate all sessions on the current client.
1import { useClerk } from "@clerk/clerk-react";23const { signOut } = useClerk();45// Use signOut to sign-out all active sessions.6await signOut();
1// Use signOut to sign-out all active sessions.2await window.Clerk.signOut();